JSON: advanced features - filtering a list & returning a value
How to find an item in a returned JSON list and select it.
Overview
You have an item that is returned in a JSON list but rather than being in a specific location in the list i.e. foo.[2].bar - it could be in any location in the list. Using a filter to identify the item, and then extracting that as a variable is how we handle this scenario.
Summary
The Assertion checks syntax uses a cut-down version of the Jinja templating library to parse the data, which allows for some filters (marked by |
) and tests as well as the direct dot-notation syntax.
For example, consider the following JSON:
{
"headers": [
{
"key": "Content-Type",
"value: "application/json"
},
{
"key": "Interaction-Id",
"value: "123456789123456789"
},
{
"key": "X-APImetrics-Id",
"value": "asdfasdfasdfasdf"
}
]
}
In this JSON, the headers are in a list. If that list is always in the same order, you can use the regular notation to find the value associated with the key Interaction-Id:
headers[1].value
However, if this list is not in a guaranteed order, you need to use filters and tests to get the value you require.
In the above example, to get the Interaction-Id, you'd use the following assertion:
headers|selectattr("key", "eq", "Interaction-Id")|map(attribute="value")|first
To explain:
- the
selectattr
filters the list of header objects where the object has an attributekey
, that is equal toInteraction-Id
- this will reduce the headers list to the a list containing the one matching item - the
map
with attribute extracts the value for the filtered list - this will leave a list with one item that is the value we want - the
first
returns the first item in the list
Filters available
More information on the filters is available in the Jinja templating library documentation. A selection of useful filters include:
Filter | Description | Parameters |
---|---|---|
first | Returns the first item in a list | |
join | Joins a list into a string, e.g. list|join(", ") creates a comma separated list | String to join values with attribute - attribute to use from list objects |
last | Returns the last item in a list | |
length | Returns the length of a list | |
map | Applies a filter on a sequence of objects or looks up an attribute. This is useful when dealing with lists of objects but you are really only interested in a certain value of it. | attribute - attribute to look up from list objects |
reject | Filters a sequence of objects by applying a test to each object, and rejecting the objects with the test succeeding. | Test for filter (see below) |
rejectattr | Filters a sequence of objects by applying a test to the specified attribute of each object, and rejecting the objects with the test succeeding. | Attribute to look up from list objects Test for filter (see below) |
replace | Return a copy of the value with all occurrences of a substring replaced with a new one. | |
select | Filters a sequence of objects by applying a test to each object, and only selecting the objects with the test succeeding. | Test for filter (see below) |
selectattr | Filters a sequence of objects by applying a test to the specified attribute of each object, and only selecting the objects with the test succeeding. | Attribute to look up from list objects Test for filter (see below) |
sort | Sort an iterable | reverse - reverse sort case_sensitive - whether sorting is case sensitive attribute - attribute to sort by |
unique | Returns a list of unique items from the the given iterable. | case_sensitive - whether sorting is case sensitive attribute - attribute to sort by |
Tests available
Tests are used as part of the filters. More information on the tests is available in the Jinja templating library documentation. A selection of useful tests include:
Test | Description |
---|---|
eq, equalto, == | Equal to |
ge, >= | Greater or equal to |
gt, > | Greater than |
le, <= | Less than or equal to |
lt, < | Less than |
ne, != | Not equal to |
none | Returns true if the variable is null |
undefined | Returns true if the variable does not exist |
Use Case
In this example, we have a sample returned JSON list where we want to find the Interaction ID and extract that as a variable for use in another call.
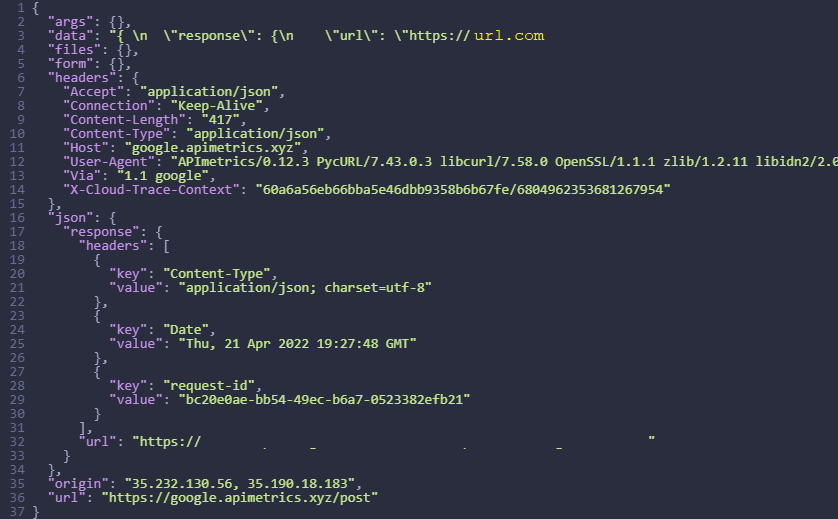
The first step is to set up an assertion check to create a filter and check that the item exists.
We do this in the 'assertions' section of the API edit pane.
json.headers|selectattr("key", "eq", "Interaction-Id")|map(attribute="value")|first
We set the ASSERTION to test it exists using the filter string in the test, thus:

We then add the command to EXTRACT the value: First we set the search criteria to find the value Interaction id.
json.headers|selectattr("key", "eq", "Interaction-Id")|map(attribute="value")|first
And then set the EXTRACT conditions to set the variable you use.

Your assertions section of the edit pane should look something like this:
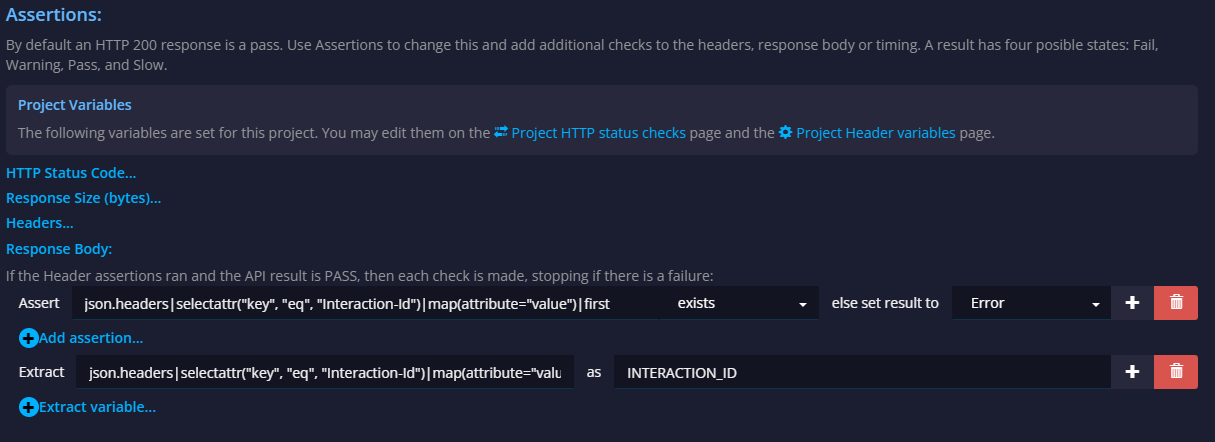
When the API call is run, the variable INTERACTION_ID is set to the value of the Interaction id in the returned JSON.
This can be used in another call in a workflow using %%INTERACTION_ID%% or INTERATION_ID
Updated almost 3 years ago